# http://inkarlslab.blogspot.com
# 09/16/2011
#
# A Demonstration of some simple MIPS instructions
# used to test QtSPIM.
#
#
#
# ori rt, rs, imm - Puts the bitwise OR of register rs and the
# zero-extended immediate into register rt => This is a
# trick for placing a constant into register rt.
#
# add rd, rs, rt - Puts the sum of registers rs and rt into register rd.
#
# sub rd, rs, rt - Puts difference of registers rs and rt into register rd.
#
# syscall - Register $v0 contains the number of the system
# call provided by QtSPIM - when $v0 is loaded with the value '10',
# this causes program to exit.
#
# It calculates 25 + 12, and 25 - 12.
#
.globl main # Make main global so you can refer to
# it by name in QtSPIM.
.text # This line tells the computer this is the
# text section of the program
# (No data contained here).
main: # Program actually starts here.
ori $t2, $0, 25 # Register $t2 gets 25
ori $t3, $0, 12 # Register $t3 gets 12
add $t4, $t2, $t3 # Register $t3 gets 25 + 12
sub $t5, $t2, $t3 # Register $t5 gets 25 - 12
ori $v0, $0, 10 # Sets $v0 to "10" so when syscall is executed, program will exit.
syscall # Exit.
This program loads constant values into two temporary registers $t2 and $t3, using the ori command.
Here is a handy list of MIPS-32 commands:
http://www.mrc.uidaho.edu/mrc/people/jff/digital/MIPSir.html
The MIPS-32 Register file contains 32 registers - each is 32 bits long. Here is a list of them and how they're used:
http://msdn.microsoft.com/en-us/library/ms253512%28v=vs.80%29.aspx
Now, back to the code:
The contents in $t2 and $t3 are added and that value gets stored in another register, $t4. Then the contents of $t3 are subtracted from the contents of $t2, then stored in $t5. After all the work is done, register $v0 is set to "10", which is the code for "EXIT". Then the program exits.
Here, in a nutshell, is how to quickly write a MIPS-32 program and run it in QtSPIM:
1) Write your program in a similar style as I have above. You can do this in any text editor. Save the file as YOUR_FILENAME.asm .
2) Start QtSPIM. Under the "simulator" menu, select "clear registers". You should see some values "zero out" in the "register window" along the left-hand side of the QtSPIM panel.
3) Under the "File" menu, select "load file". A file browser window will pop up - locate your .asm file and select it. The program should load into QtSPIM.
4) Under the "Simulator" menu, select "Run Parameters". Enter "0x00400024" - without the quotation marks, into the box entitled "address or label to start running program". This simply tells QtSPIM which line your code starts on, since there is other initialization code that appears whenever you start QtSPIM. That code ends with a syscall at address 0x00400020.
5) Under the "Registers" menu, select "decimal". This will display the values in your register as normal base 10 (decimal) numbers, which helps us to easily verify that the math is being done according to design.
6) Using the single-step button seen in the second picture below, step through the program. You can also use "F10" on your keyboard - you may find this more convenient. Each time you click the button, note what happens to the values in each of the registers in the left hand window, as well as the program counter.
Note, too, that both the program counter and the register address increments by 4; this makes sense because a MIPS-32 register is 32 bits, or 4 bytes, long. Gotta love it when real-world experience actually matches what's in the book :)
The sequence of pictures below show the program as I stepped through it.
Ready, get set ...
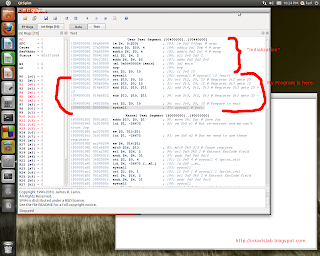
Now, we start stepping through the program:
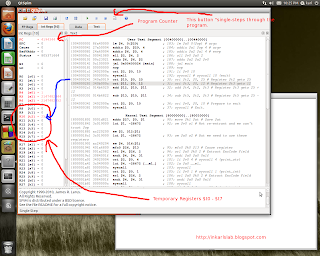
As we step, the "ori" instructions load constants into the registers in preparation for some simple math.
Addition completed:
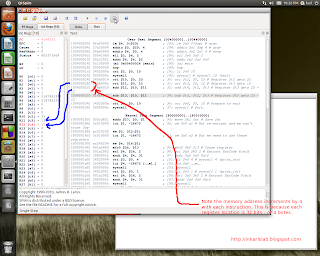
Now, the subtraction just completed:
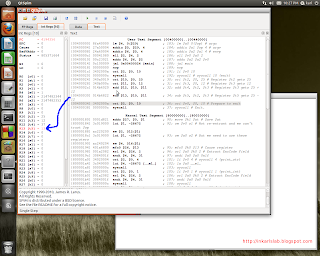
Program finished running:
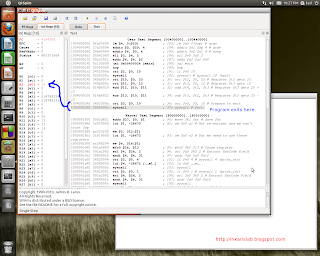
I would like to add that the creator of SPIM, Jim Larus, was of great help in answering my questions and emails during the installation of QtSPIM on my Fedora box. Mr. Larus is a true gentleman and has created a really cool and useful application - and I wanted to voice my appreciation for his efforts here.
sooo helpful thanks for the tutorial
ReplyDeleteI'm very happy I could help out!
ReplyDeleteKarl
I am thrilled to have found your post. This is the kind of information needed in the Getting Started guide for the simulator.
ReplyDeleteThanks again!
Ethan
Glad I can help!! As complex as computers and software are, there is a desperate need for better communication and tutorials for the end users. I strive to help others when I learn about related stuff.
Delete